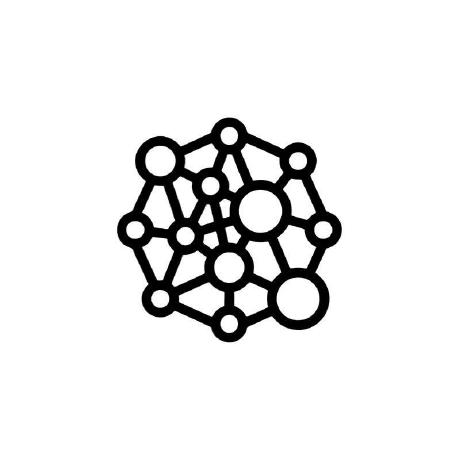
How to Use
To use the agentis-framework, follow these steps:
- Visit https://github.com/AgentisLabs/agentis-frameworkhttps://github.com/Agenti...
- Follow the setup instructions to create an account (if required)
- Connect the MCP server to your Claude Desktop application
- Start using agentis-framework capabilities within your Claude conversations
Additional Information
Created
March 5, 2025
Company
Start building your own MCP Server
Interested in creating your own MCP Server? Check out the official documentation and resources.
Learn More