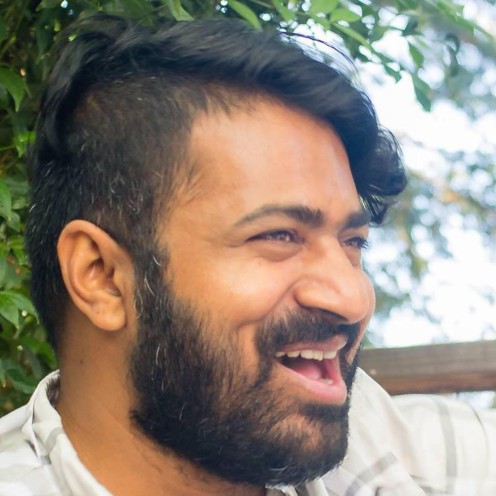
How to Use
To use the MCP NodeJS Debugger, follow these steps:
- Visit https://www.npmjs.com/package/@hyperdrive-eng/mcp-nodejs-debuggerhttps://www.npmjs.com/pac...
- Follow the setup instructions to create an account (if required)
- Connect the MCP server to your Claude Desktop application
- Start using MCP NodeJS Debugger capabilities within your Claude conversations
Additional Information
Created
March 21, 2025
Company
Start building your own MCP Server
Interested in creating your own MCP Server? Check out the official documentation and resources.
Learn More