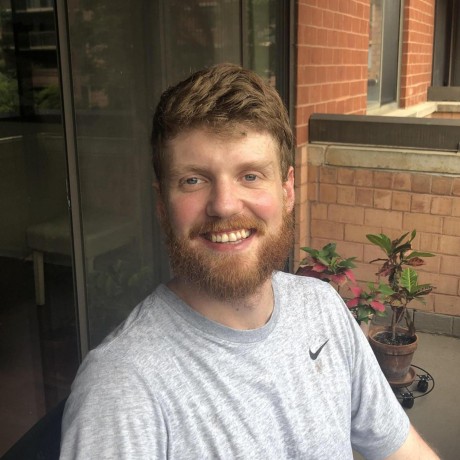
How to Use
To use the mcp-researcher-project, follow these steps:
- Visit https://github.com/nappernick/mcp-researcher-projecthttps://github.com/napper...
- Follow the setup instructions to create an account (if required)
- Connect the MCP server to your Claude Desktop application
- Start using mcp-researcher-project capabilities within your Claude conversations
Additional Information
Created
December 20, 2024
Company
Start building your own MCP Server
Interested in creating your own MCP Server? Check out the official documentation and resources.
Learn More