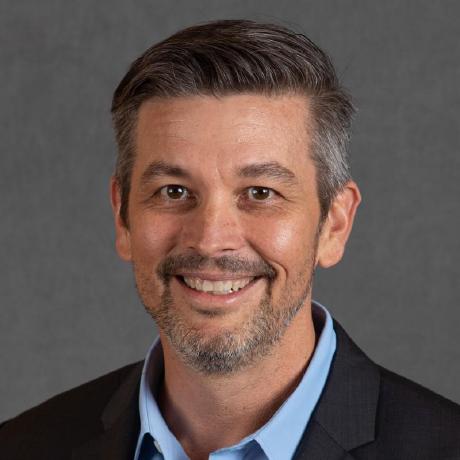
How to Use
To use the pipedrive-mcp-server, follow these steps:
- Visit https://github.com/WillDent/pipedrive-mcp-serverhttps://github.com/WillDe...
- Follow the setup instructions to create an account (if required)
- Connect the MCP server to your Claude Desktop application
- Start using pipedrive-mcp-server capabilities within your Claude conversations
Additional Information
Created
March 13, 2025
Company
Start building your own MCP Server
Interested in creating your own MCP Server? Check out the official documentation and resources.
Learn More