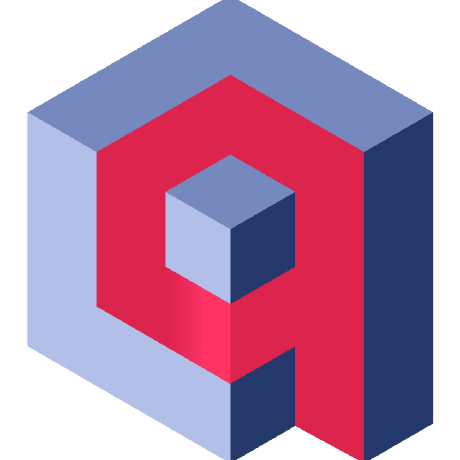
How to Use
To use the mcp-server-qdrant, follow these steps:
- Visit https://github.com/qdrant/mcp-server-qdrant/https://github.com/qdrant...
- Follow the setup instructions to create an account (if required)
- Connect the MCP server to your Claude Desktop application
- Start using mcp-server-qdrant capabilities within your Claude conversations
Additional Information
Created
March 12, 2025
Company
Related MCP Servers
Start building your own MCP Server
Interested in creating your own MCP Server? Check out the official documentation and resources.
Learn More