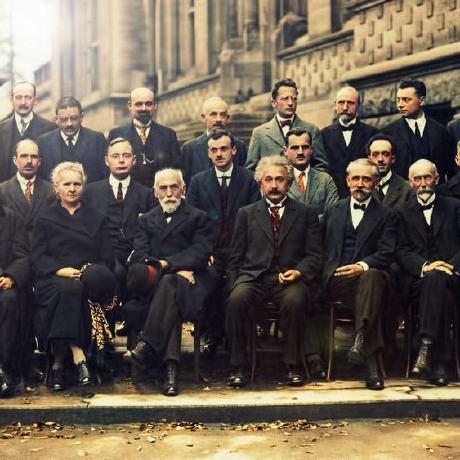
How to Use
To use the YOLO-MCP-Server, follow these steps:
- Visit https://github.com/GongRzhe/YOLO-MCP-Serverhttps://github.com/GongRz...
- Follow the setup instructions to create an account (if required)
- Connect the MCP server to your Claude Desktop application
- Start using YOLO-MCP-Server capabilities within your Claude conversations
Additional Information
Created
March 11, 2025
Company
Start building your own MCP Server
Interested in creating your own MCP Server? Check out the official documentation and resources.
Learn More